Here's How to Improve Your Data Structure and Algorithms Skills
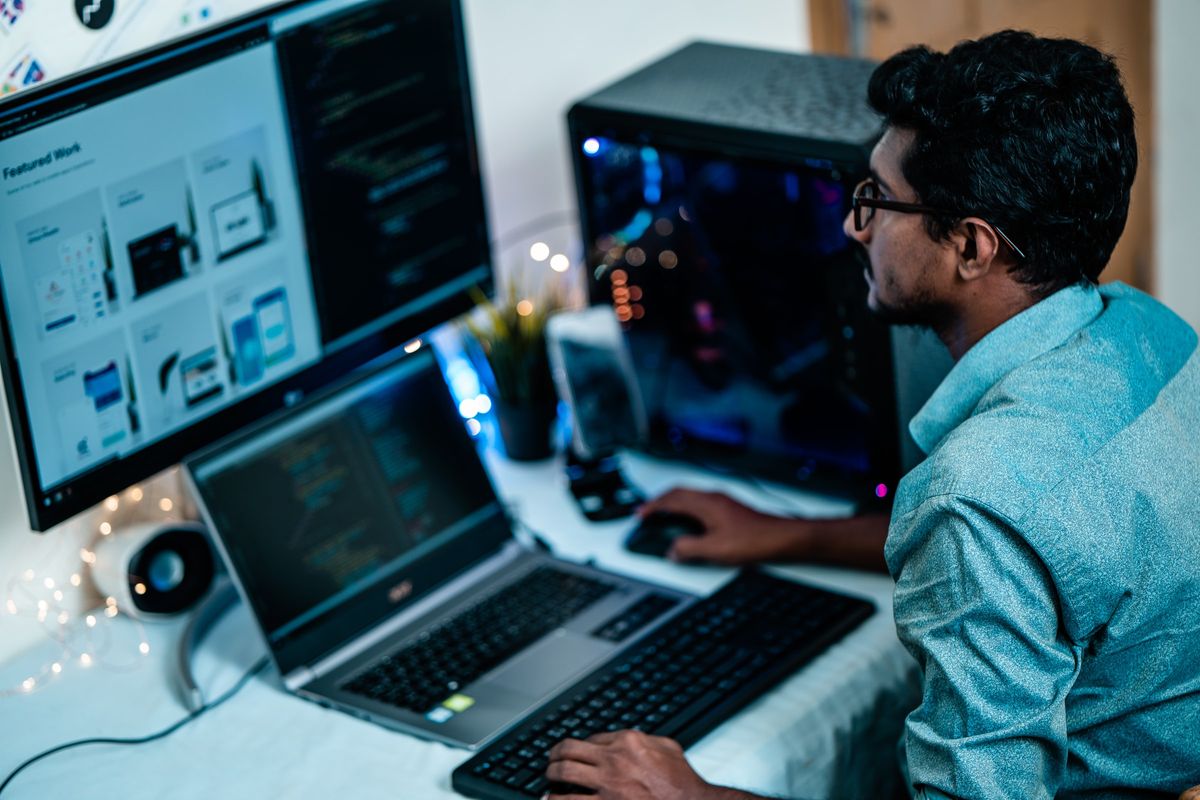
Data structure and algorithms are some of the most important skills that software engineers should have in their toolbox, especially if they want to be prepared for a job interview at a tech company.
Software engineers should consider these five steps to improve their data structure and algorithms skills.
1. Understand the fundamentals.
Brush up on the fundamentals of data structures. For example, most software engineers should be able to recognize a branch, sibling, left subtree and right subtree when discussing tree data structure.
Learn some of the most common algorithms:
- Pigeonhole Principle
- Squareroot Decomposition
- Set Theory
- Bitwise Operations
- Graph Theory
- Dynamic Programming
- Greedy Algorithms
You should also learn how data can be represented. Pay attention to the basics of digital logic design, Boolean algebra, computer arithmetic, floating-point representation and cache design. Knowing or learning the programming languages C or Assembly can help, too.
2. Work on logic.
Take some time to understand the problem statement before proceeding with logic. Often, software engineers give up when designing the logic of a program because they jump to the solutions.
Some tactics for logic-building include:
- Try a dry run on paper or a whiteboard to ensure you understand the problem and check the test cases.
- Write pseudocode and verify with the problem requirement.
- Pick out the problem’s key constraints. Understand the required time and space complexity.
- Try a brute-force approach, and then optimize it with different data structures.
- Identify the edge cases where your applied algorithm could fail.
3. Drill down on the basic algorithms.
Learning data structures isn’t enough for most software engineers. To level up as a programmer, it’s helpful to boost your algorithms skills. Mastering algorithms can make you a more competitive software engineer, as you can now optimize code for minimal execution time.
Focus on these basic algorithms:
- Graph algorithms: Breadth-first search, depth-first search, Dijkstra Algorithm, Floyd Warshall Algorithm, Kruskal and Prim’s Algorithm, and topological sorting
- Dynamic programming: Knapsack Problem, the longest common subsequence, the longest increasing subsequence, subset sum problem, and Partition Problem
- Searching and sorting: Binary search, merge and quick sort, KMP Algorithm, counting sort, and Manacher’s sorting
- Number theory: Primality test, sieve of Eratosthenes, segmented sieve, Wilson Algorithm, and Pollard Rho Algorithm
- Mathematical algorithms: Count inversions, square root decomposition, Catalan number, Hungarian Algorithm, and log exponentiation
- Geometry and network flow: Convex hull, Graham scan, lie intersection, interval tree, stable marriage problem, Fulkerson Algorithm, Edmon Karp Algorithm, and Dinic Algorithm
- Modulo algorithms: Euler Totient Algorithm, module exponentiation and Chinese Remainder Theorem
4. Focus your studies.
A common mistake many software engineers make is to target too many problems. Instead, it’s crucial to develop some depth in your problem-solving.
Pace yourself and maintain continuity as part of your coding studies. Remember: Don’t jump straight to the solution. Your goal is to improve your logic skills.
5. Revise and participate.
Data structure and algorithms study should be active. When building your skills, participate and solve questions on HackerRank or LeetCode. Active participation can give you an idea of what a real-world application might look like, including a whiteboarding job interview in tech.
Here are some tips that could help you:
- Note the particular data structure of the problem and the time and space complexities of the insertion, update and deletion operations.
- Think about how your approach might be judged. You might be judged on whether you have correct syntax, your ability to solve a problem within a specific time frame, comprehension of the problem statement, and your knowledge of the required data structure to solve the question.
- Practice online so that you are comfortable with online coding environments.
- Learn to optimize your code to improve your space and time complexity.
This article was written by Sandeep Mishra for HackerNoon and was lightly edited and published with permission.