What to Expect in a Front-End Software Engineer Job Interview
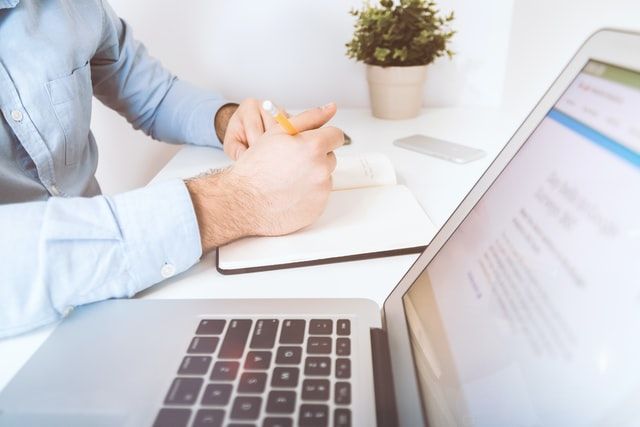
Technical job interviews can be challenging, especially when you have to think, solve and analyze problems while someone watches. However, job interviews are just another skill you can master with the right information and practice.
Every company has a different job interview style and might require a custom strategy, but in the end, most front-end software engineer job interviews have a similar structure. These are the most common topics and questions you might get asked as a front-end developer.
What are the most common front-end software engineer job interview questions?
Front-end software engineers should know JavaScript, even if your day-to-day work is in Angular, React or Vue. You should know the basics of JavaScript.
Be prepared to answer some of these most common technical job interview questions:
- What is an event loop?
- What is a promise?
- What is the “this” keyword? (You should be able to answer closure and context questions.)
- What is the difference between an arrow and a regular function?
- How do you implement Array.prototype.map or Array.prototype.reduce?
- What is the debounce function? Can you implement it?
- What is the throttle function? Can you implement it?
- What does an inheritance allow you to do?
Do you have to study algorithms for a front-end software engineer job interview?
While it is unlikely you’ll ever need to traverse a binary tree while working as a front-end software engineer, algorithm questions are common in technical job interviews. Large tech companies, such as Amazon, Apple, Google, Meta or Microsoft, might ask some algorithm problems in their front-end software engineer job interviews.
What are the most common algorithms problems for front-end software engineer job interviews?
The most common algorithms problems in front-end software engineer technical job interviews are:
- Array or object-related questions
- Breadth-first search or depth-first search questions (traversing a tree)
- Linked lists
For example, you might be asked:
- Reverse a string.
- Write a function to check if a word is a palindrome.
- Write a function that solves a maze. You will typically be given start coordinates, and your work must return whether you got out of the maze successfully.
- Reverse a linked list. Find the n-th node from the end of the linked list.
How to approach algorithms problems in a front-end software engineer technical job interview
Algorithms problems are typically no different than how you might approach any technical job interview, even as a front-end software engineer. The top six tips for approaching algorithms problems for a front-end software engineer are:
- Ask questions. Don’t rush to solve the problem in a technical job interview.
- Talk aloud and go through your work. You don’t necessarily have to reach a perfect and working solution in a technical job interview.
- Start with a plan or pseudocode. You want to show the job interviewer that you like to plan and be methodical in your work.
- Don’t panic. Start with the simple things: inputs and outputs. Focus on edge cases.
- Pay attention to efficiency. If you understand that your code complexity could be better, acknowledge it aloud. Work with the job interviewer to reach a better solution.
- Practice before the technical job interview. It is very rare for software engineers to write code on a whiteboard or piece of paper. Get used to the format, so you have one less thing to worry about during your job interview.
Front-end software engineer job interview take-home assignment tips
Some employers might require you to build a simple app using a specific framework or library, such as Angular, React or Vue. You might have to do the assignment before the on-site job interview or as a part of the on-premise technical job interview. It could be a good idea to ask if you can bring your laptop to the on-site job interview to use your familiar environment and machine.
You will most likely be given a public API to work through in a front-end software engineer assignment. The job interviewer wants to understand how you write actual code, so consider these best practices:
- Keep your code clean. Indent, pay attention to variable names, and remove unused code.
- Use the best practices of the assigned framework.
- Don’t forget to debounce requests sent to the server, prevent server calls when fewer than three characters are typed, or take care of edge cases, such as missing information in the API.
- Pay attention to CSS. Get comfortable using Flexbox.
The bottom line
As a front-end software engineer, your first instinct might be to focus on technical challenges and coding problems. However, you are still taking part in a job interview. You will need to talk about your past experience, professional interests and challenges, and the details of the features, products or code you shipped.
This article was written by Sveta Slepner for HackerNoon and was lightly edited and published with permission.